A comprehensive guide to RESTful APIs
Welcome to my comprehensive guide on RESTful APIs – your ultimate resource for untangling the complexities of Representational State Transfer (REST) Application Programming Interfaces (API). In this article, we will delve into this API’s definitions, characteristics, advantages, limitations, examples, and FAQs. Whether you’re a seasoned developer, a tech enthusiast, or a business professional, this article is for you. Let’s dive in.
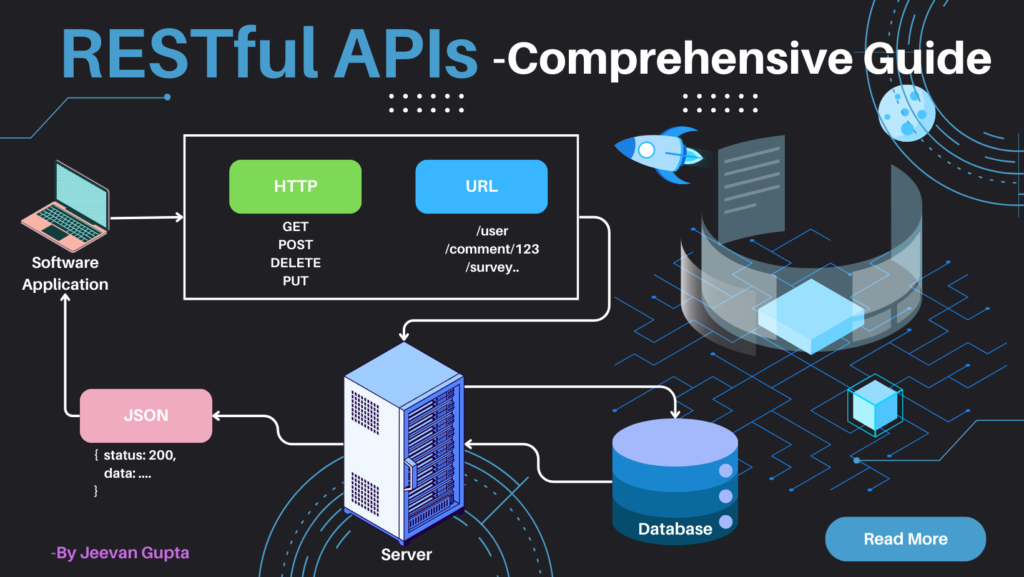
What is an API?
An API stands for “Application Programming Interface”, a set of rules and protocols that allows different software applications (components) to communicate with each other. It defines the methods and data formats that applications can use to request and exchange information. APIs act as bridges, enabling seamless integration between diverse systems, services, or platforms, and facilitating the sharing of data and functionality. APIs play a crucial role in modern software development by enabling developers to leverage existing functionalities without having to understand the internal workings of the systems they interact with or reinvent the wheel.
What are RESTful APIs?
RESTful APIs or Representational State Transfer APIs, are a popular type of web API that adhere to REST architectural principles. REST relies on a client/server approach that separates the front and back ends of the API and provides considerable flexibility in development and implementation.
They are based on the concept of resources, which are identified by unique URLs, and can be manipulated using HTTP methods such as GET, POST, PUT, and DELETE. This APIs are known for their simplicity, scalability, and compatibility with different programming languages and platforms.
What is REST?
REST stands for Representational State Transfer is a software architecture that imposes conditions on how an API should work. Its flexibility allows for easy implementation and modifications, ensuring high performance and reliable communication at scale. REST-based architecture brings visibility and cross-platform portability to any API system.
Rest was introduced by Roy Fielding in his doctoral dissertation in 2000. Fielding, a computer scientist and one of the principal authors of the HTTP specification developed REST as a set of principles to guide the design of scalable and maintainable network architectures.
API developers have various architectural options, and when opting for the REST style, the resulting APIs are termed REST APIs. Correspondingly, web services adopting REST architecture are known as RESTful web services. Although commonly referred to as RESTful web APIs, the terms REST API and RESTful API are interchangeable. This architecture provides a versatile and efficient approach to designing APIs that seamlessly integrate with diverse systems.
Characteristics of RESTful APIs
Resource-Based: In these APIs, resources (such as data entities or services) are identified by unique URIs (Uniform Resource Identifiers). These resources can be manipulated using standard HTTP methods.
Stateless: RESTful APIs are stateless, meaning that each request from a client to a server contains all the information needed to understand and fulfill the request. RESTful APIs do not store any client state, making them highly scalable and facilitating load balancing.
Uniform interface: RESTful APIs provide a uniform and consistent way to interact with resources, promoting simplicity and ease of use. This includes conventions like using standard HTTP methods and resource URIs.
Cacheable: RESTful APIs support the caching of responses, which improves performance and reduces server load.
Self-descriptive: RESTful APIs provide metadata and hypermedia links within responses, enabling clients to discover and navigate the API’s capabilities.
Representation: Resources in RESTful APIs are represented in a format, often JSON or XML, making it easy for clients and servers to understand and exchange data.
How RESTful APIs work
As mentioned earlier RESTful APIS follows client-server architecture. The client contacts the server by using the API to request data and the server responds with the requested data.
Here are the broad steps:
Step 1: The client initiates a request
A client application, such as a web browser or mobile app, initiates a request to a RESTful API server. This request is typically made over HTTP (Hypertext Transfer Protocol), the standard protocol for communication on the World Wide Web.
Step 2: Request includes HTTP method and resource URI:
The request includes the HTTP method, which specifies the desired action to be performed on a resource. Common HTTP methods include GET (retrieve data), POST (create new data), PUT (update existing data), and DELETE (remove data).
The request also includes a Uniform Resource Identifier (URI), which identifies the requested resource. For example, a URI might be https://example.com/users/123, which would request data about the user with ID 123.
Step 3: Server processes the request:
The RESTful API server receives the request and processes it. The server will validate the request, ensure that the client has the necessary authorization to access the resource and perform the requested action.
Step 4: Server sends a response:
The RESTful API server sends a response back to the client. The response includes an HTTP status code, which indicates whether the request was successful (e.g., 200 OK) or not (e.g., 404 Not Found).
The response also includes the requested data, if applicable. The data is typically formatted in a structured format, such as JSON or XML.
Step 5: Client receives the response:
The client application receives the response from the RESTful API server. The client will parse the response and use the data to update its user interface or perform other actions.
Advantages and limitations of RESTful APIs
Advantages of RESTful APIs:
- Simplicity: Easy to understand and use.
- Scalability: Can handle increased demand efficiently.
- Flexibility: Allows for easy modifications and updates.
- Compatibility: Works well across different devices and platforms.
- Statelessness: Simplifies communication, enhancing reliability.
Limitations of RESTful APIs:
- Limited Functionality: This may not support every complex operation.
- Overhead in Large Transactions: Can be slower in handling extensive requests.
- Security Concerns: Requires additional measures for robust security.
- Limited Real-time Support: Not ideal for applications requiring instant updates.
- Dependency on Internet Standards: Relies on specific web standards for operation.
Example of Popular RESTful APIs
- Twitter API: Allows developers to interact with Twitter’s functionalities, such as posting tweets, searching for tweets, and retrieving user information.
- Google Maps API: Empowers developers to integrate maps and location-based services into their applications, enabling features like geocoding, directions, and place search.
- Spotify API: Spotify provides a RESTful API that grants access to music data, playlists, and user profiles, empowering developers to integrate music-related features into their applications.
- OpenWeatherMap API: Description: OpenWeatherMap’s RESTful API offers weather data, forecasts, and current conditions, enabling developers to integrate weather information into their applications.
- Stripe API: Description: Stripe’s REST API is used for online payment processing, allowing developers to integrate secure and customizable payment solutions into e-commerce platforms.
- GitHub API: Facilitates interactions with the GitHub platform, enabling developers to manage repositories, create issues, and perform various other actions programmatically.
Programmatic Example – Python code to make RESTful API call
Syntax/Layout:
import requests # Replace the URL with the actual endpoint you want to call api_url = "https://api.example.com/data" # Define any required parameters or headers params = {'param1': 'value1', 'param2': 'value2'} headers = {'Authorization': 'Bearer YOUR_ACCESS_TOKEN'} # Make a GET request to the API endpoint response = requests.get(api_url, params=params, headers=headers) # Check if the request was successful (status code 200) if response.status_code == 200: # Parse and work with the response data (assuming JSON data for simplicity) data = response.json() print("API Response:", data) else: # Print an error message if the request was not successful print("Error:", response.status_code, response.text)
Practical Example 1:
import requests # Replace with your OpenWeatherMap API key api_key = "YOUR_API_KEY" # City name to get weather data for city_name = "London" # Base URL for the OpenWeatherMap API base_url = "https://api.openweathermap.org/data/2.5/weather" # Complete URL with city name and API key url = f"{base_url}?q={city_name}&appid={api_key}" # Send GET request to the API response = requests.get(url) # Check if the request was successful if response.status_code == 200: # Convert the JSON response to a Python dictionary data = response.json() # Extract relevant weather information weather_description = data["weather"][0]["description"] temperature = data["main"]["temp"] # Print the extracted information print(f"Weather in {city_name}: {weather_description}") print(f"Temperature: {temperature:.2f}°C") else: print("Error: Unable to fetch weather data")
FAQs
Q1: What is a RESTful API?
Answer: A RESTful API (Representational State Transfer) is a type of web API that adheres to the principles of REST architecture. It uses standard HTTP methods for communication and is widely used for building scalable and efficient web services.
Q2: How does REST differ from SOAP?
Answer: REST is an architectural style based on a stateless client-server model, using standard HTTP methods, while SOAP (Simple Object Access Protocol) is a protocol with a more rigid structure, often using XML for communication. REST is generally considered more lightweight and easier to implement.
Q3: What are the key characteristics of RESTful APIs?
Answer: Key characteristics include statelessness, resource-based identification, representation of resources in formats like JSON or XML, a uniform interface, and standard HTTP methods (GET, POST, PUT, DELETE).
Q4: What is the role of URIs in RESTful APIs?
Answer: URIs (Uniform Resource Identifiers) uniquely identify resources in a RESTful API. They serve as addresses for resources, enabling clients to interact with specific entities on the server.
Q5: How does statelessness in RESTful APIs work?
Answer: Statelessness means that each client request to the server must contain all the information needed to understand and fulfill that request. The server doesn’t store any information about the client’s state between requests, leading to a more scalable and reliable system.
Q6: Can RESTful APIs use protocols other than HTTP?
Answer: While RESTful principles were originally designed with HTTP in mind, it’s possible to implement RESTful concepts with other protocols. However, due to its ubiquity, HTTP remains the most common choice for RESTful APIs.
Q7: What are the common data formats used in RESTful APIs?
Answer: JSON (JavaScript Object Notation) and XML (eXtensible Markup Language) are the most common data formats used for representing resources in RESTful APIs. JSON is favored for its simplicity and ease of use.
Q8: How does versioning work in RESTful APIs?
Answer: API versioning is typically handled by including a version number in the URI or using custom headers. This ensures that changes to the API can be managed without breaking existing client implementations.
Q9: What is HATEOAS in the context of RESTful APIs?
Answer: HATEOAS (Hypermedia As The Engine Of Application State) is a principle of RESTful APIs that suggests including hypermedia links in the API responses. These links guide clients on the possible actions they can take, making the API more self-descriptive.
Q10: How can security be implemented in RESTful APIs?
Answer: Security measures in RESTful APIs include using HTTPS for encrypted communication, implementing authentication mechanisms (such as API keys or OAuth), and validating user input to prevent common security vulnerabilities like SQL injection or cross-site scripting (XSS).
Summary
In the interconnected world of today, data exchange is the lifeblood of digital interactions. Application Programming Interfaces (APIs) have emerged as the cornerstone of seamless communication between various software applications and services. Among the diverse API landscapes, RESTful APIs stand out as a dominant architectural style, shaping the way data is accessed, manipulated, and shared across the web.
This comprehensive guide delves into the world of RESTful APIs, providing a simplified yet informative overview of this essential technology. From understanding the fundamental concepts of APIs and REST to exploring the characteristics, workings, advantages, and limitations of RESTful APIs, this guide equips you with the knowledge and understanding necessary to harness the power of RESTful APIs.
If you like this article and think it was easy to understand and might help someone you know, do share it with them. If you want my help, you can reach out to me through this Contact Form to discuss your specific needs and requirements. Thank You! See you soon.
For any suggestions or doubts ~ Get In Touch
Checkout out my other API Integration and Setup Guide