How to get accessible Google Ads accounts or customer details using Google Ads API and python?
Hi Everyone, hope you are keeping well. Thank you so much for stopping by. Today we are going to see how to develop a python code to extract Google Ads account or customer details using Google Ads API and python. This article will demonstrate two ways to get Google Ads Account details, especially Account ID.
To give the overall idea of these two options – First, directly get it from the Google Ads account dashboard, the second way is to use python code and Google Ads to get Ads Account ID and the rest of the Ads account details.
Why is Google Ads Account ID important?
To successfully query Google Ads API and get performance data or account data from Google Ads API the most important parameter we need to pass/provide is Google Ads Account ID. Without this, a query made through Google Ads API fails since it has no idea which accounts to query and return the data.
Resource for using Google Ads API (AdWord API) :
Let’s Get Started …
Method1: Getting Ads Account ID from Google Ads
First, log in to Google Ads. On successful login, you will see a screen to select a Google Ads Account. If not the only option you have is to create an account.

If you have one or more google ads accounts you will be asked to select one. You can get an idea from the below screen.

To get the Account ID of an account. The 10-digit number shown at the side of each account in the above screengrab is the account id of that account. One will get the same account id from the dashboard.
Below is the screen grab of the Google Ads dashboard. The account id is on the right side along with the account name and user login.
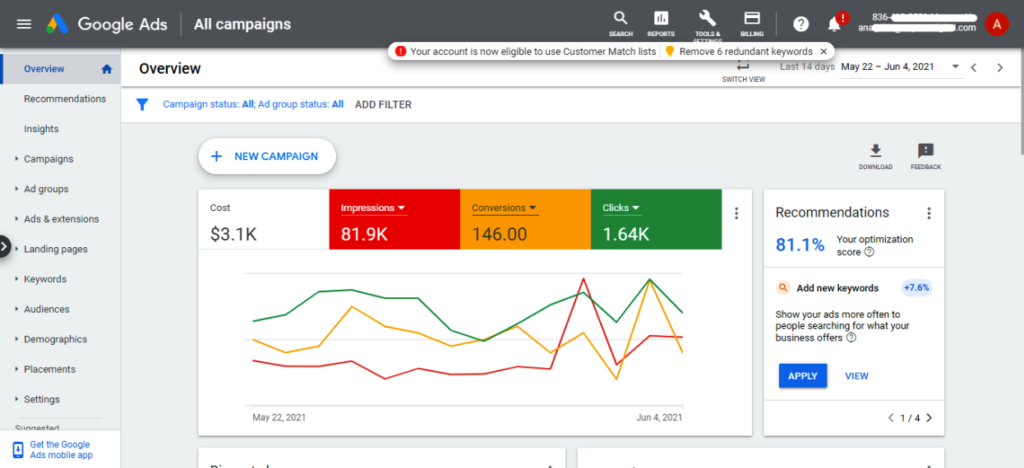
Method 2: Python code to get Google Ads Account Details
In this method, we will build python logic to get Google Ads Account ID and other details (like location, currency, etc). Python code will be using the Google Ads API (formerly AdWord API). Hence it’s important you have completed the Google Ads API setup steps and you have all the tokens to create the below credential file.
2.1- Create a JSON file to store Tokens
Before requesting the Google Ads Account details using API we need to OAuth authentication ourselves. To do this easily we will be creating a JSON file, which will store all authentication-related credentials -like Client ID, Client Secret, Access Token, Refresh Token, and Developer Token.
Creating a JSON file to store credentials makes it easy to maintain, update and track credentials as needed. This practice also provides security to your credentials from other team members or one can easily exclude this file from keeping it in the public repository. Proceed by saving the below JSON as “google_ads_cred.json”.
{ "client_id":"Replace with Client Id", "client_secret":"Replace with Client Secret", "access_token":"Replace with Access Token", "refresh_token":"Replace with Refresh Token", "developer_token" :"Replace with Developer Token", "version": "v10" }
2.2- Building Python Logic to get Account ID and Account details
Here, we will first read the credentials from the above JSON file (line number: 64 – 72). Next, we are going to define a function google_ads_authentication (line number: 11 – 29) to OAuth authenticate on behalf of the user. Will be calling this function at line number 74 along with passing credentials that need to authenticate.
Now we will define a function google_ads_account (line number: 31 – 55) to make the Google Ads API call and get Ads Account details in the response. We will complete this project by calling the above function (line number: 76).
Consider going through the code, and try to get a basic understanding of what’s going on. Don’t forget to save the code file as “google_ads_accounts.py”.
#!/usr/local/bin/python3 #python3 ./get_google_ads_accounts.py import sys import json import time from datetime import datetime import pandas as pd from google.ads.googleads.client import GoogleAdsClient def google_ads_authentication(token): try: google_ads_client = GoogleAdsClient.load_from_dict(token) print("\nFunction (google_ads_authentication) - authentication process finished successfully ") return google_ads_client except: print("\n*** Function (google_ads_authentication) Failed *** ",sys.exc_info()) def get_google_ads_customer(client): customer_service = client.get_service("CustomerService") customer_resource_names = (customer_service.list_accessible_customers().resource_names) customer_id_list = [] googleads_service = client.get_service("GoogleAdsService") for customer_resource_name in customer_resource_names: customer_id = googleads_service.parse_customer_path(customer_resource_name)["customer_id"] customer_id_list.append(customer_id) #print("\n customer_id_list : ",customer_id_list) query = """ SELECT customer_client.client_customer, customer_client.level, customer_client.manager, customer_client.descriptive_name, customer_client.currency_code, customer_client.time_zone, customer_client.id, customer_client.test_account FROM customer_client WHERE customer_client.level <= 1 and customer_client.test_account = 'false' """ #and customer.test_account != TRUE PARAMETERS include_drafts=true final_list=[] for c in customer_id_list: try: response = googleads_service.search(customer_id=str(c), query=query) acc = None for d in response: customer_client = d.customer_client #print("\ncustomer detail : ",customer_client) if customer_client.level == 0: if acc is None: acc = customer_client tmp = {"id":acc.id,"name":acc.descriptive_name, "time_zone":acc.time_zone} final_list.append(tmp) # else: # print("\n level not 0 ",customer_client) except: pass account_dt = pd.DataFrame(final_list) return account_dt if __name__ == '__main__': try: timestamp = datetime.strftime(datetime.now(),'%Y-%m-%d : %H:%M') print("Get Google Ads account details process Started at ",datetime.now()) start = time.process_time() #Read the Credentials from the JSON file. cred_file = "./google_ads_cred.json" cred_data = open(cred_file, 'r') google_ads_cred = json.load(cred_data) client = google_ads_authentication(google_ads_cred) accounts_details_df = get_google_ads_customer(client) print("\n Account Details :\n",accounts_details_df) print("\nGet Google Ads account details process ends at ",datetime.now()) print("Processing Time : ",(time.process_time()-start)) except: print("\n *** Get Google Ads account processing Failed :", sys.exc_info())
Run above file with command -> python3 ./google_ads_accounts.py
You should see a tabular output giving you Account Details along with your Account ID.
Hope this guide was able to explain both the method of getting a Google Ads Account and made it simple to understand, especially to get the Account details by building a python code using Google Ads API.
Hope I was able to solve the problem. If you like this article and think it was easy to understand do share it with your friends and connection. Thank you! see you soon.
If you have any questions or comments feel free to reach me at ->
Checkout out my other API Integration and Coding Solution Guide