How to get LinkedIn Ads Account Details or LinkedIn profile using LinkedIn Access Token and Python?
Greetings, everyone! I appreciate your visit. Today, we’ll delve into the world of Python scripting and the LinkedIn API to extract valuable LinkedIn Ads Account details or LinkedIn Ads Profile information. This guide focuses on obtaining LinkedIn Ads Account IDs and Ad Account Names, crucial for fetching LinkedIn Campaign data using Python and the LinkedIn API. Whether you’re new to this or looking to enhance your skills, this code can be your gateway to unlocking LinkedIn Ads Account details tailored to your specific needs.
There are two methods to obtain LinkedIn Ads Account details, particularly the Account ID. You can either directly retrieve it from the LinkedIn Advertising account dashboard, or you can opt for a more automated approach using Python code and the LinkedIn API, specifically the LinkedIn Advertising API. Both methods have their advantages, and we will explore them in detail.
Let’s get started …
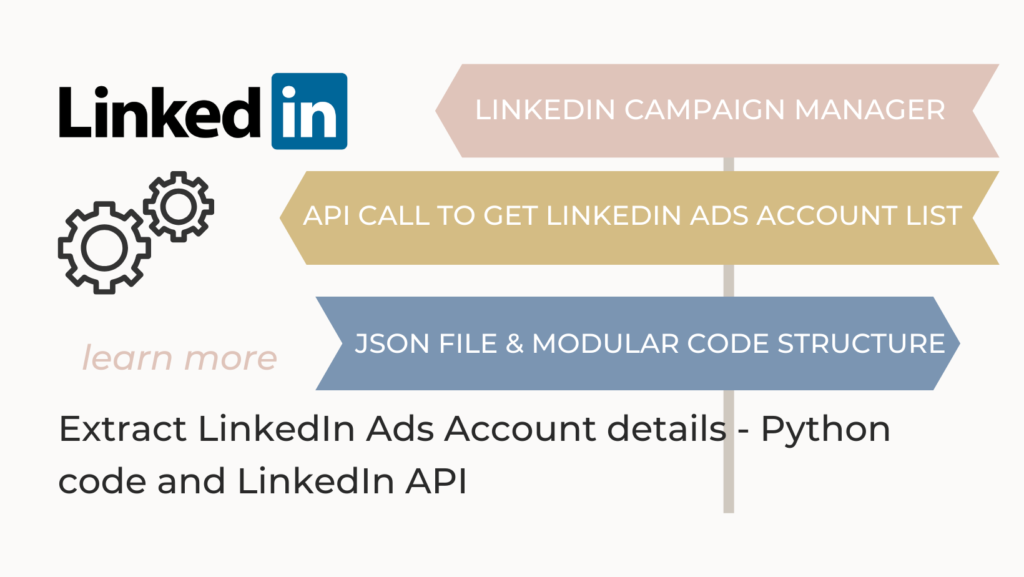
What is the LinkedIn API?
The LinkedIn Marketing API is used to programmatically query data, create and manage ads, create and manage campaigns, get account-related data, and perform a wide variety of other tasks. This guide helps you complete all the steps needed to use LinkedIn Marketing API successfully.
The LinkedIn API is the REST API and is the heart of all programmatic interactions with LinkedIn. LinkedIn API enables you to bring the power of the world’s largest professional network to your apps. By using the LinkedIn API (also known as LinkedIn Marketing API) you can use several LinkedIn services. Most importantly you can extract your campaign performance data using this API.
Table of Contents:
- Getting Ads Account ID from LinkedIn Campaign Manager
- Python code to get LinkedIn Ad Account Details (Ad Account ID)
Quick Access:
Method 1: Getting Ads Account ID from LinkedIn Campaign Manager
First log in to LinkedIn Campaign Manager. On successful login, you will see a list of Linkedin Ads Accounts you can manage.
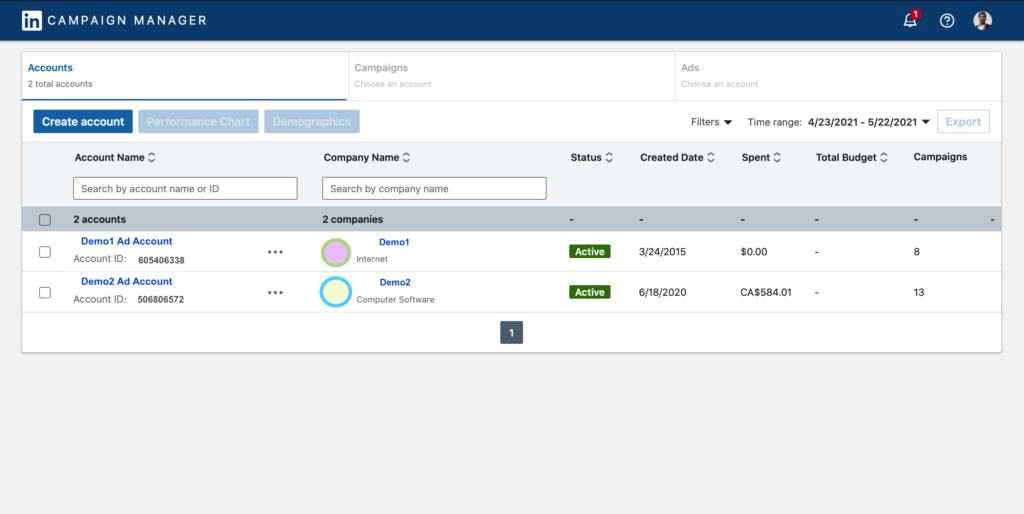
Where is the LinkedIn Ads account id?
As you can see from the above image 2 LinkedIn Ads accounts can be managed. The Ads account ID is specified just after “Account ID:”.
From our example, the Linkedin Ads Account ID for Ad account “Demo1” is 605406338 and for Ad account “Demo2” is 506806572.
Method 2: Python code to get LinkedIn Ad Account Details (Ad Account ID)
In this method, we will be building Python logic to get LinkedIn Ads Account ID along with other Details (like location, currency, etc). Python code will be using the LinkedIn API. Hence you must complete the LinkedIn API setup steps and you have all the tokens to create the below credential file.
2.1- Create a JSON file to store Tokens
Before requesting the LinkedIn Ads Account details using LinkedIn API we need to OAuth authentication ourselves. To do this easily we will be creating a JSON file, which will store all authentication-related credentials -like Client ID, Client Secret, Access Token, Refresh Token, and Developer Token.
Creating a JSON file to store credentials makes it easy to maintain, update, and track credentials as needed. This practice also provides security to your credentials from other team members or one can easily exclude this file from keeping it in the public repository. Proceed by saving the below JSON as “linkedin_cred.json”.
{ "client_id":"Replace with Client ID", "access_token":"Replace with Access Token ", "refresh_token":"Replace with Refresh Token", "client_secret" : "Replace with Client Secret" }
2.2- Building Python Logic to get Account ID
Here, we will first read the credentials from the above JSON file (line number: 46 – 49). Next, we are going to define a function (line number: 9 – 37) to make the LinkedIn API call and get Ads Account details in the response. The last one calls the above function (line number: 52).
Consider going through the code, and try to get a basic understanding of what’s going on. Don’t forget to save the code file as “linkedin_ads_accounts.py”.
#!/usr/bin/python3 #command to run the code: python3 ./linkedin_ads_account.py import requests import json import pandas import sys from datetime import datetime, timedelta def get_linkedin_ads_account(access_token): try: ver = "v2" url = "https://api.linkedin.com/v2/adAccountsV2?q=search&search.type.values[0]=BUSINESS&search.status.values[0]=ACTIVE" headers = {"Authorization": "Bearer "+access_token} #make the http call r = requests.get(url = url, headers = headers) #define a data frame to store the Linkedin account details account_df = pandas.DataFrame(columns=["account_id","account_name"]) if r.status_code != 200: print("\n ### something went wrong ### ",r) else: response_dict = json.loads(r.text) if "elements" in response_dict: accounts = response_dict["elements"] for acc in accounts: tmp_dict = {} account_id = acc["id"] account_name = acc["name"] account_currency = acc["currency"] tmp_dict["account_id"] = account_id tmp_dict["account_name"] = account_name tmp_dict["account_currency"] = account_currency account_df = account_df.append(tmp_dict,ignore_index = True) return account_df except: print("\n*** get_linkedin_ads account funtion Failed *** ",sys.exc_info()) raise if __name__ == '__main__': try: timestamp = datetime.strftime(datetime.now(),'%Y-%m-%d : %H:%M') print("DATE : ",timestamp,"\n") print("LinkedIn Ads Account data extraction process Starts") #loading and reading crdentials from JSON file. cred_file = "./linkedin_cred.json" linkedin_cred= open(cred_file, 'r') cred_json = json.load(linkedin_cred) access_token = cred_json["access_token"] #call authentication function accounts_details_df = get_linkedin_ads_account(access_token) print("\n Account details :\n",accounts_details_df) print("\nLinkedin Ads Account data extraction process Finished") except: print("\n*** Linkedin Ads Account data extraction processing Failed *** ", sys.exc_info())
Run the above file with the command -> python3 ./linkedin_ads_accounts.py
You should see an output giving you Account Details along with your Account ID.
Hope this guide was able to explain both the method of getting a LinkedIn Ads Account ID and made it simple to understand, especially to get the Account ID by building a Python code using LinkedIn API.
Hope I was able to solve the problem. If you like this article and think it was easy to understand do share it with your friends and connection. Thank you! See you soon.
If you have any questions or comments feel free to reach me at ->
Checkout out my other API Integration and Coding Solution Guide