Understanding the Python Dictionary – Common Interview Questions, Tips and Tricks
Welcome to the fascinating world of Python dictionaries! Whether you’re a seasoned developer or just starting your coding journey, understanding Python dictionaries is a crucial skill that can elevate your programming prowess. In this blog post, we’ll unravel the complexities of Python dictionaries together, exploring common interview questions, handy tips, and tricks that will not only boost your confidence but also enhance your overall coding experience. If you don’t know – what is Python programming language? its usage and benefit then I recommend you to go through all about Python programming language guide. So, buckle up as we embark on a journey to demystify the power of Python dictionaries, making your code more efficient and your programming journey even more enjoyable! Let’s dive in.
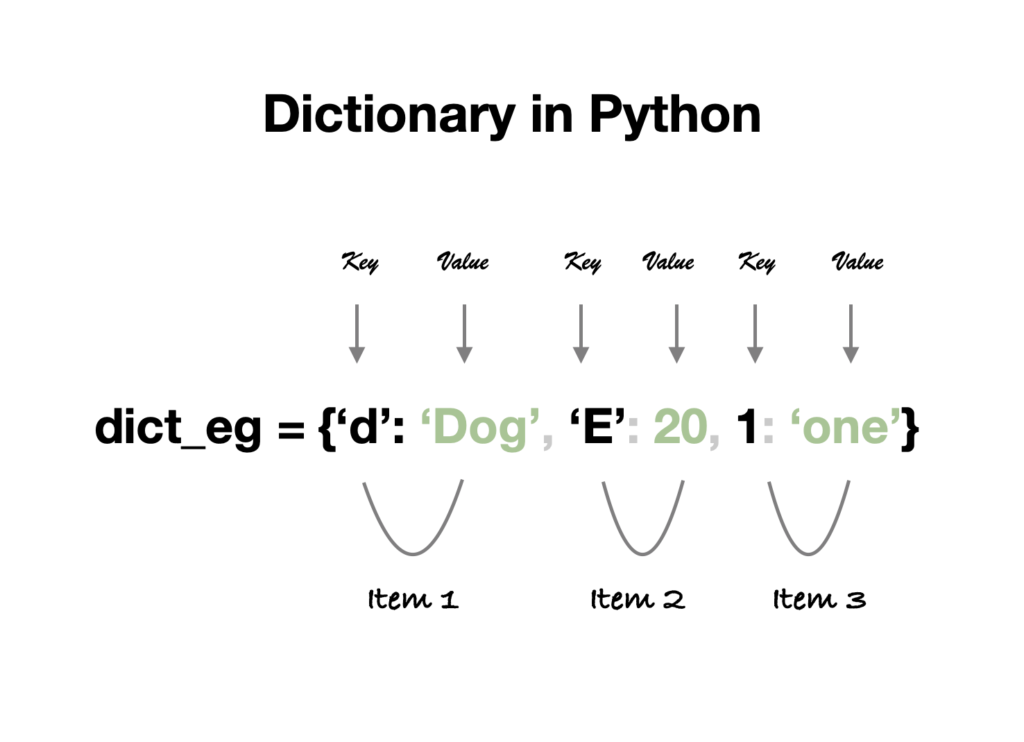
What is a Python Dictionary?
A Python dictionary is a powerful data structure used to store collections of items in an organized way. Unlike lists, which store items in a specific order based on their index, dictionaries store items in key-value pairs.
Here’s a breakdown of its key features:
Key-value pairs: Each item in a dictionary consists of a unique key and its associated value. Keys act like labels or identifiers, while values represent the actual data you want to store. Think of it like a phonebook, where names (keys) map to phone numbers (values).
Unordered (Python 3.6 and earlier) vs. Ordered (Python 3.7 and later): In older versions of Python (before 3.7), dictionaries were unordered, meaning the order of items wasn’t guaranteed. However, from Python 3.7 onwards, dictionaries maintain the order in which items were added, which can be useful for certain situations.
Mutable: You can modify the values associated with keys after creating a dictionary. You can even add or remove key-value pairs entirely, making dictionaries very flexible for storing and managing data.
Key restrictions: Keys must be immutable data types like strings, numbers, or tuples. This means they cannot be changed after they’re assigned. This ensures efficient lookups and prevents conflicts within the dictionary.
Use cases: Dictionaries are incredibly versatile and used in various scenarios, including:
- Storing user information: Websites and applications often use dictionaries to store user data like names, email addresses, and preferences.
- Mapping words to definitions: Dictionaries are ideal for creating dictionaries (like the one you’re using now!), where words (keys) map to their meanings (values).
- Configuration files: Applications can use dictionaries to store configuration settings in key-value pairs for easy modification.
- Data analysis: Grouping and analyzing data based on categories or attributes is often done using dictionaries.
Python Dictionary Syntax
dictionary = {key1:value1,key2:value2}
Table of Contents:
- Creating a dictionary.
- Reading dictionary item/value.
- Add key/value to the dictionary.
- Edit the key name in the dictionary.
- Update the value in the dictionary.
- Delete/clean/empty a dictionary.
- Remove or delete an item from a dictionary.
- Filter a dictionary.
- Concatenate multiple dictionaries.
- Sum all values/elements of a Dictionary.
- Multiply all items’ values in the dictionary.
- Convert a dictionary object into a string.
- Printing dictionaries.
- Printing Python dictionary in a table format.
- Maximum value dictionary item.
- Minimum value dictionary item.
- Sorting dictionary items by value.
How to initialize, declare or create a Python dictionary?
As we mentioned before, the syntax of the dictionary is {key: value}. You can use literal to initialize dictionaries.
Example:
#!/usr/local/bin/python3 #declaring dictionary with literal or data dict1 = {'A': 2, 'B': 5, 'C': 4} print("\nDictionary eg1 : ",dict1) dict2 = {'D': 'Dog', 'E': 20, 'F': 4.8} print("\nDictionary eg2 : ",dict2)
We can also declare an empty dictionary object by using an inbuilt function dict(). Constructor dict is also a type conversion function. We can pass parameters to the dict function and create a dictionary, without parameter dict() create an empty dictionary.
Example:
#declaring empty dictionary using constructor dict1 = dict() print("\nEmpty Dictionary: ",dict1) #dict() can also be used to create dictionary by passing argument dict2 = dict(A=1, B='birth', C= 6.3) print("\n Dictionary dict2 : ", dict2)
Do remember, a tuple can also be converted to a dictionary using Python type conversion.
How to traverse a dictionary or iterate over a dictionary?
There are many ways to iterate over a dictionary, will understand three of them below.
- Iterate through all keys.
- Iterate through all values.
- Iterate through all key-value pairs.
Examples:
#!/usr/local/bin/python3 #Lets create a dictionary dict1 # that will have keys as states and value as Abbreviation dict1 = {'Goa': 'GA', 'Assam': 'AS', 'Karnataka': 'KA', 'Maharashtra': 'MH', 'Odisha': 'OR'} print("\nState and its abbreviation Dictionary is -> ",dict1) # Iterating over keys print("\n States are :") for k in dict1: print(k) #Iterating over all values print("\n Abbreviation are:") for ab in dict1.values(): print(ab) #Iterating through key, value pairs print("\n States and its Abbreviation are:") for k,v in dict1.items(): print(k," : ",v)
Output:
State and its abbreviation Dictionary is -> {'Goa': 'GA', 'Assam': 'AS', 'Karnataka': 'KA', 'Maharashtra': 'MH', 'Odisha': 'OR'} Goa Assam Karnataka Maharashtra Odisha Abbreviation are: GA AS KA MH OR States and its Abbreviation are: Goa : GA Assam : AS Karnataka : KA Maharashtra : MH Odisha : OR
How to add key and value to a Python dictionary?
We can add new key-value pairs by using subscription notation. This method adds a new key value to the dictionary if the key doesn’t exist and if the key exists, the current value gets overwritten by the new value.
Example:
#!/usr/local/bin/python3 #Lets create a dictionary dict1 # that will have keys as states and value as Abbreviation dict1 = {'Goa': 'GA', 'Karnataka': 'KA', 'Maharashtra': 'MH'} print("\nOriginal Dictionary -> ",dict1) #Using Subscription notation #syntax: Dictionary_Name[New_Key_Name] = New_Key_Value dict1['Assam'] = 'AS' dict1['Tamil Nadu'] = 'TN' print("\nDictionary after adding 2 items -> ",dict1)
Output:
Jeevan-Gupta:Python Tutorial jeevangupta$ python3 ./dictionary_operation_tutorial\ copy.py Original Dictionary -> {'Goa': 'GA', 'Karnataka': 'KA', 'Maharashtra': 'MH'} Dictionary after adding 2 items -> {'Goa': 'GA', 'Karnataka': 'KA', 'Maharashtra': 'MH', 'Assam': 'AS', 'Tamil Nadu': 'TN'}
How to change the name of a key in a dictionary?
There are two ways to change the name key of a dictionary. First, add a new key that will have the value of the old and then delete the old key. The second way is to use pop. Pop is the built-in function of Python to remove the last or given index/key. So we remove the old key and put the value back into the dictionary with the new key name.
Example:
#change name of key in dictionary #way1 dict1['Odisha'] = dict1['Tamil Nadu'] del dict1['Tamil Nadu'] print("\nDictionary after key name change -> ",dict1) #way2 dict1['Chhattisgarh'] = dict1.pop('Maharashtra') print("\nDictionary after key name change -> ",dict1)
Output:
Original Dictionary -> {'Goa': 'GA', 'Karnataka': 'KA', 'Maharashtra': 'MH', 'Assam': 'AS', 'Tamil Nadu': 'TN'} Dictionary after key name change -> {'Goa': 'GA', 'Karnataka': 'KA', 'Maharashtra': 'MH', 'Assam': 'AS', 'Odisha': 'TN'} Dictionary after key name change -> {'Goa': 'GA', 'Karnataka': 'KA', 'Assam': 'AS', 'Odisha': 'TN', 'Chhattisgarh': 'MH'}
How do update values in a dictionary?
The value of keys in a dictionary can be updated by using the update function of python. Update takes one parameter without a parameter; it doesn’t make any changes to the dictionary. The parameter can be a dictionary with {OldKey: NewValue} – where the key should already exist in the dictionary and the value is the new value you want to add in place of the old value. Parameters also can be tuples like OldKey= NewValue. Another way to update a value in a dictionary is using the subscription method i.e. dict[key]=NewValue.
Example:
#updating value in dictionary #using update() tmp={"Chhattisgarh":"CT"} dict1.update(tmp) print("\nDictionary after key value update -> ",dict1) #OR dict1.update(Chhattisgarh = "CT") print("\nDictionary after key value update -> ",dict1) #using subscription notation dict1["Odisha"] = "OR" print("\nDictionary after key value update -> ",dict1)
Output:
Original Dictionary -> {'Goa': 'GA', 'Karnataka': 'KA', 'Assam': 'AS', 'Odisha': 'TN', 'Chhattisgarh': 'MH'} Dictionary after key value update -> {'Goa': 'GA', 'Karnataka': 'KA', 'Assam': 'AS', 'Odisha': 'TN', 'Chhattisgarh': 'CT'} Dictionary after key value update -> {'Goa': 'GA', 'Karnataka': 'KA', 'Assam': 'AS', 'Odisha': 'TN', 'Chhattisgarh': 'CT'} Dictionary after key value update -> {'Goa': 'GA', 'Karnataka': 'KA', 'Assam': 'AS', 'Odisha': 'OR', 'Chhattisgarh': 'CT'}
How to delete/clean/empty out a dictionary?
A dictionary can be deleted or cleaned or emptied by using a clear method. Clear() is the Python inbuilt method and it doesn’t take any parameters. Another way is to assign an empty dictionary to a dictionary, but this is not emptying the dictionary, it’s assigning an empty dictionary to the existing dictionary. When we clear a dictionary, the actual dictionary content is removed due to which all references i.e. other dictionaries created from this dictionary become empty.
Example:
#delete/clean/empty a dictionary dict1 = {'Goa': 'GA', 'Karnataka': 'KA', 'Maharashtra': 'MH', 'Chhattisgarh': 'CT'} print("\n\nOriginal Dictionary -> ",dict1) #using clear method dict2 = dict1 # dict2 is the Reference of dict1 print("\nReference Dictionary (befor)-> ",dict2) dict1.clear() print("Dictionary -> ",dict1) print("Reference Dictionary (after)-> ",dict2) #VS dict1 = {'Goa': 'GA', 'Karnataka': 'KA', 'Maharashtra': 'MH', 'Chhattisgarh': 'CT'} dict2 = dict1 # dict2 is the Reference of dict1 print("\nReference Dictionary (befor)-> ",dict2) dict1 = {} print("Dictionary -> ",dict1) print("Reference Dictionary (after)-> ",dict2)
Output:
Original Dictionary -> {'Goa': 'GA', 'Karnataka': 'KA', 'Maharashtra': 'MH', 'Chhattisgarh': 'CT'} Reference Dictionary (befor)-> {'Goa': 'GA', 'Karnataka': 'KA', 'Maharashtra': 'MH', 'Chhattisgarh': 'CT'} Dictionary -> {} Reference Dictionary (after)-> {} Reference Dictionary (befor)-> {'Goa': 'GA', 'Karnataka': 'KA', 'Maharashtra': 'MH', 'Chhattisgarh': 'CT'} Dictionary -> {} Reference Dictionary (after)-> {'Goa': 'GA', 'Karnataka': 'KA', 'Maharashtra': 'MH', 'Chhattisgarh': 'CT'}
How to remove or delete an item (i.e. key: value pair) from a dictionary?
A dictionary item can be deleted in two ways. First using the pop() method. pop() is the Python inbuilt method and it takes one parameter that is the key that is needed. pop delete the key and value associated with it from the dictionary.
The second way is using the del keyword (NOTE: del is not a function or method in Python; it’s a keyword in Python that is used to delete any object in Python). So del can also be used to delete key values from a dictionary. Examples of both ways are shown below.
Example:
#delete item from dictionary based on key dict1 = {'Goa': 'GA', 'Karnataka': 'KA', 'Maharashtra': 'MH', 'Chhattisgarh': 'CT'} print("\nOriginal Dictionary -> ",dict1) #using pop() dict1.pop("Maharashtra") print("\nDictionary -> ",dict1) #using del() del dict1["Chhattisgarh"] print("\nDictionary -> ",dict1)
Output:
Original Dictionary -> {'Goa': 'GA', 'Karnataka': 'KA', 'Maharashtra': 'MH', 'Chhattisgarh': 'CT'} Dictionary -> {'Goa': 'GA', 'Karnataka': 'KA', 'Chhattisgarh': 'CT'} Dictionary -> {'Goa': 'GA', 'Karnataka': 'KA'}
How to filter Python dictionaries based on value or/and key?
Sometimes we need to get only some items from the dictionary and not. This is quite common in web development where we require to get only a selective dictionary item and show it on UI.
One requirement is to get an item from the dictionary based on a given value. For example, get all smartphones that have a battery capacity of more than then 3500 mAh. A result is a dictionary too.
Example:
#!/usr/local/bin/python3 #Lets create a dictionary named as smart_phone_dict # which contain smartphone name and its battery capacity smart_phone_dict = {'Samsung Galaxy F22': 5500, 'Realme X7 Max': 4000, 'Moto G10 Power': 3000, 'OnePlus 8 Pro':5000, 'Samsung Galaxy M32': 3500} print("\nOriginal Dictionary -> ",smart_phone_dict) #filter dictionary based on Value #get item i.e key:value from dictionary where mAh is >= 3500 #Way 1 result = {} for key in smart_phone_dict: value = smart_phone_dict[key] if value >= 3500: tmp = {key:value} result.update(tmp) print("Way 1 : ",result) #Way 2 (optimised) result = {key:value for (key, value) in smart_phone_dict.items() if value >= 3500} print("Way 2 : ",result)
Output:
Original Dictionary -> {'Samsung Galaxy F22': 5500, 'Realme X7 Max': 4000, 'Moto G10 Power': 3000, 'OnePlus 8 Pro': 5000, 'Samsung Galaxy M32': 3500} Way 1 : {'Samsung Galaxy F22': 5500, 'Realme X7 Max': 4000, 'OnePlus 8 Pro': 5000, 'Samsung Galaxy M32': 3500} Way 2 : {'Samsung Galaxy F22': 5500, 'Realme X7 Max': 4000, 'OnePlus 8 Pro': 5000, 'Samsung Galaxy M32': 3500}
Another requirement is to filter dictionaries based on key. For example, get the smartphone details of the smartphone (key) is “OnePlus 8 Pro”. Another problem can be getting all the smartphones of the brand “Samsung”. NOTE: add the below code to the above code.
Example:
# #filter dictionary based on Key #get item i.e key:value from dictionary where smart phone name is "OnePlus 8 Pro" result = {key:value for (key, value) in smart_phone_dict.items() if key == "OnePlus 8 Pro"} print("\nsmartphone name filter result : ",result) #get item i.e key:value from dictionary where smart phone brand name is "Samsung" result = {key:value for (key, value) in smart_phone_dict.items() if "Samsung" in key} print("smartphone brand filter result : ",result)
Output:
Output: smartphone name filter result : {'OnePlus 8 Pro': 5000} smartphone brand filter result : {'Samsung Galaxy F22': 5500, 'Samsung Galaxy M32': 3500}
How to concatenate multiple Python dictionaries into one?
There are several ways to concatenate a dictionary into one dictionary. Let’s see each of them through an example.
Let’s create two dictionaries ->
#example to demonstrate ways of concatenating dictionary #Lets create a dictionary named as smart_phone_dict1 # which contain smartphone name and its battery capacity smart_phone_dict1 = {'Samsung Galaxy F22': 5500, 'Realme X7 Max': 4000, 'Moto G10 Power': 3000, 'OnePlus 8 Pro':5000, 'Samsung Galaxy M32': 3500} print("\nOriginal Dictionary 1 -> ",smart_phone_dict1) #Lets create another dictionary named as smart_phone_dict2 # which contain smartphone name and its battery capacity smart_phone_dict2 = {'iPhone 13 Pro Max': 4373, 'iPhone 12 Pro Max': 3733} print("Original Dictionary 2 -> ",smart_phone_dict2)
Option 1: dictionary concatenation using update() function.
#dictionary concatenation using python update() function smart_phone_dict1.update(smart_phone_dict2) print("\nWay 1 Concatenate_dict : ",smart_phone_dict1)
Option 2: dictionary concatenation using a single expression i.e. **.
#dictionary concatenation using ** [double star] i.e single expression concatenate_dict = {**smart_phone_dict1, **smart_phone_dict2} print("\nWay 2 Concatenate_dict : ",concatenate_dict)
Option 3: dictionary concatenation as per Python3.9 i.e. using the “|” operator
#dictionary concatenation using | operator concatenate_dict = smart_phone_dict1 | smart_phone_dict2 print("\nWay 3 Concatenate_dict : ",concatenate_dict)
Output:
Original Dictionary 1 -> {'Samsung Galaxy F22': 5500, 'Realme X7 Max': 4000, 'Moto G10 Power': 3000, 'OnePlus 8 Pro': 5000, 'Samsung Galaxy M32': 3500} Original Dictionary 2 -> {'iPhone 13 Pro Max': 4373, 'iPhone 12 Pro Max': 3733} Way 1 Concatenate_dict : {'Samsung Galaxy F22': 5500, 'Realme X7 Max': 4000, 'Moto G10 Power': 3000, 'OnePlus 8 Pro': 5000, 'Samsung Galaxy M32': 3500, 'iPhone 13 Pro Max': 4373, 'iPhone 12 Pro Max': 3733} Way 2 Concatenate_dict : {'Samsung Galaxy F22': 5500, 'Realme X7 Max': 4000, 'Moto G10 Power': 3000, 'OnePlus 8 Pro': 5000, 'Samsung Galaxy M32': 3500, 'iPhone 13 Pro Max': 4373, 'iPhone 12 Pro Max': 3733} Way 3 Concatenate_dict : {'Samsung Galaxy F22': 5500, 'Realme X7 Max': 4000, 'Moto G10 Power': 3000, 'OnePlus 8 Pro': 5000, 'Samsung Galaxy
How to sum all values or elements of a dictionary?
The sum of all the dictionary values can be found in 2-3 ways. Let’s understand each of them through examples.
Create a dictionary ->
# #example to demonstrate ways of summing up dictionary values #Lets create a dictionary named as smart_phone_dict # which contain smartphone name and its battery capacity smart_phone_dict = {'Samsung Galaxy F22': 5500, 'Realme X7 Max': 4000, 'Moto G10 Power': 3000, 'OnePlus 8 Pro':5000, 'Samsung Galaxy M32': 3500} print("\nOriginal Dictionary -> ",smart_phone_dict1)
Approach 1: using built-in sum() function.
#approch1 : sum() mAh_list = [] for i in smart_phone_dict: mAh_list.append(smart_phone_dict[i]) mAh_sum = sum(mAh_list) print("Approach 1 mAh_sum : ",mAh_sum)
Approach 2: using for loop and values() function.
#Approach 2 : Using For loop to iterate through values using values() function mAh_sum = 0 for i in smart_phone_dict.values(): mAh_sum = mAh_sum + i print("Approach 2 mAh_sum : ",mAh_sum)
Approach 3: Use a for loop to iterate through items in the dictionary.
#Approach 3 : Using For loop to iterate through items of dictionary mAh_sum = 0 for i in smart_phone_dict: mAh_sum = mAh_sum + smart_phone_dict[i] print("Approach 3 mAh_sum : ",mAh_sum)
Output:
Original Dictionary -> {'Samsung Galaxy F22': 5500, 'Realme X7 Max': 4000, 'Moto G10 Power': 3000, 'OnePlus 8 Pro': 5000, 'Samsung Galaxy M32': 3500, 'iPhone 13 Pro Max': 4373, 'iPhone 12 Pro Max': 3733} Approach 1 mAh_sum : 21000 Approach 2 mAh_sum : 21000 Approach 2 mAh_sum : 21000
How to multiply all items’ values in the Python dictionary?
This task is pretty similar to finding the sum of values. Here we are going to see 3 ways to get the product of dictionary values.
# #example to demonstrate ways of multiplying dictionary values #Lets create a dictionary named as smart_phone_dict # which contain smartphone name and its battery capacity smart_phone_dict = {'Samsung Galaxy F22': 5500, 'Realme X7 Max': 4000, 'Moto G10 Power': 3000, 'OnePlus 8 Pro':5000, 'Samsung Galaxy M32': 3500} print("\nOriginal Dictionary -> ",smart_phone_dict1)
Approach 1: Using numpy.prod() function:
#approch1 : numpy.prod() to get the multiplications import numpy mAh_list = [] for i in smart_phone_dict: mAh_list.append(smart_phone_dict[i]) mAh_mul = numpy.prod(mAh_list) print("Approach 1 mAh_mul : ",mAh_mul)
Approach 2: Using for loop and values() function:
#Approach 2 : Using For loop to iterate through values using values() function mAh_mul = 1 for i in smart_phone_dict.values(): mAh_mul = mAh_mul * i print("Approach 2 mAh_mul : ",mAh_mul)
Approach 3: Use a for loop to iterate through items in the dictionary and * operator.
#Approach 3 : Using For loop to iterate through items of dictionary mAh_mul = 1 for i in smart_phone_dict: mAh_mul = mAh_mul * smart_phone_dict[i] print("Approach 3 mAh_mul : ",mAh_mul)
Output:
Original Dictionary -> {'Samsung Galaxy F22': 5500, 'Realme X7 Max': 4000, 'Moto G10 Power': 3000, 'OnePlus 8 Pro': 5000, 'Samsung Galaxy M32': 3500, 'iPhone 13 Pro Max': 4373, 'iPhone 12 Pro Max': 3733} Approach 1 mAh_mul : 1155000000000000000 Approach 2 mAh_mul : 1155000000000000000 Approach 3 mAh_mul : 1155000000000000000
How to convert a Python dictionary object into a string?
A dictionary can be converted to string using several ways some of the common approaches are listed below with examples for each.
One way is to use the Python inbuilt function str() to convert a dictionary or any other data type to a string. Another way to convert a dictionary to a string is using the JSON.dumps() function.
Example:
# #Lets create a dictionary named as india_state_dict # that will have keys as states and value as Abbreviation india_state_dict = {'Goa': 'GA', 'Karnataka': 'KA', 'Maharashtra': 'MH', 'Chhattisgarh': 'CT'} print("\nOriginal Dictionary -> ",india_state_dict) #approch1 : using str() function string_version_dict = str(india_state_dict) print ("\nData type : ", type(string_version_dict)) print ("Result string : ", string_version_dict) #approch2 : using json.dumps() function import json string_version_dict = json.dumps(india_state_dict) print ("\nData type : ", type(string_version_dict)) print ("Result string : ", string_version_dict)
Output:
Original Dictionary -> {'Goa': 'GA', 'Karnataka': 'KA', 'Maharashtra': 'MH', 'Chhattisgarh': 'CT'} Data type : <class 'str'> Result string : {"Goa": "GA", "Karnataka": "KA", "Maharashtra": "MH", "Chhattisgarh": "CT"} Data type : <class 'str'> Result string : {'Goa': 'GA', 'Karnataka': 'KA', 'Maharashtra': 'MH', 'Chhattisgarh': 'CT'}
Different ways of Python printing dictionaries?
A dictionary can be printed in a single row or line by line. To print dictionary elements line by line one can come up with several codes. The below examples show the 2 most common ways to print dictionaries line by line.
Example:
# #Lets create a dictionary named as india_state_dict # that will have keys as states and value as Abbreviation india_state_dict = {'Goa': 'GA', 'Karnataka': 'KA', 'Maharashtra': 'MH', 'Chhattisgarh': 'CT'} print("\nDirect print of Original Dictionary -> ",india_state_dict) #line by line print dictionary item using list comprehension [print(key,':',value) for key, value in india_state_dict.items()] print("\n") #line by line print of dictionary by iterating over keys for key in india_state_dict: print(key," : ",india_state_dict[key])
Output:
Direct print of Original Dictionary -> {'Goa': 'GA', 'Karnataka': 'KA', 'Maharashtra': 'MH', 'Chhattisgarh': 'CT'} Goa : GA Karnataka : KA Maharashtra : MH Chhattisgarh : CT Goa : GA Karnataka : KA Maharashtra : MH Chhattisgarh : CT
Printing Python dictionary in a table format.
Example:
#print dictionary as table print("\n{:<8} {:<15}".format('State','Abbreviation')) for k, v in india_state_dict.items(): print("{:<8} {:<15}".format(k, v))
Output:
State Abbrevation Goa GA Karnataka KA Maharashtra MH Chhattisgarh CT
Get an item corresponding to the max value from a dictionary
To get an item (say the key name) of max value in a dictionary, there are several ways. Let’s understand each of these methods with examples.
#!/usr/local/bin/python3 #Lets create a Dictionary dict1 dict = {'GA':100, 'AS':34, 'KA':150, 'MH':70} print("\nDictionary is -> ",dict)
We can get max value keys using values() method, keys() method and max() function.
# Python code to find key with Maximum value in Dictionary #method1 - using keys() method, values() methods and max() function # store values of dictionary in list object dict_val dict_val = list(dict.values()) # store keys of dictionary in list object dict_keys dict_keys = list(dict.keys()) print(dict_keys[dict_val.index(max(dict_val))])
Another way to get the max value key is using the lambda and max() function.
#method 2 - using lambda and max() function. res = max(dict, key= lambda x: dict[x]) print(res)
One more way to achieve the above requirement is using the operator module and max() function.
#method 3 - using operator module and max() function import operator res = max(dict.items(), key = operator.itemgetter(1))[0] print(res)
NOTE: You can run the above example by copying the entire code into a single Python file. All the above methods should give you the same output i.e KA
Get an item corresponding to min value from a dictionary?
In order to get find an item having a minimum value in a dictionary, there are three common ways. Let’s understand each of these code logic with examples.
#!/usr/local/bin/python3 #Lets create a Dictionary dict1 dict = {'GA':100, 'AS':34, 'KA':150, 'MH':70} print("\nDictionary is -> ",dict)
We can get min value keys using values() method, keys() method and min() function.
# Python code to find key with Maximum value in Dictionary #method1 - using keys() method, values() methods and max() function # store values of dictionary in list object dict_val dict_val = list(dict.values()) # store keys of dictionary in list object dict_keys dict_keys = list(dict.keys()) print(dict_keys[dict_val.index(min(dict_val))])
Another way to get the min value key is using the lambda and min() function.
#method 2 - using lambda and min() functions. res = min(dict, key= lambda x: dict[x]) print(res)
One more way to achieve the above requirement is using the operator module and min() function.
#method 3 - using operator module and min() function import operator res = min(dict.items(), key = operator.itemgetter(1))[0] print(res)
NOTE: You can run the above example by copying the entire code into a single Python file. All the above methods should give you the same output i.e. AS.
Sort a dictionary by value
Dictionary items can be sorted based on values. This can be achieved in three ways. Let’s understand each of these methods with examples.
#!/usr/local/bin/python3 #Lets create a Dictionary dict1 dict = {'GA':100, 'AS':34, 'KA':150, 'MH':70} print("\nDictionary is -> ",dict)
We can get min value keys using values() method, keys() method and sorted() function.
# Python code to sort dictionaries by value. #method 1 - using values() method, keys() method and sorted() function. # store values of dictionary in list object dict_val dict_val = list(dict1.values()) # store keys of dictionary in list object dict_keys dict_keys = list(dict1.keys()) res = {dict_keys[dict_val.index(v)]:v for v in sorted(dict_val)} print(res)
Another way to get the sorted items is using the lambda and sorted() function.
#method 2 - using lambda and sorted() function. res = {k:v for k,v in sorted(dict1.items(), key=lambda item: item[1])} print(res)
The Last one is to use the operator module and sorted() function to sort dictionary items.
#method 3 - using operator module and sorted() function import operator res = {k:v for k,v in sorted(dict1.items(), key = operator.itemgetter(1))} print(res)
NOTE: You can run the above example by copying the entire code into a single Python file. All the above methods should give you the same output i.e {‘AS’: 34, ‘MH’: 70, ‘GA’: 100, ‘KA’: 150}
Hope I have made it easy to understand the Python dictionary and its basic operations.
If you have any questions or comments feel free to reach me at.