Understanding the Set Data Type in Python: Common Interview Questions, Tips, and Tricks
Hi there! Hope you are doing well. Welcome back, in this article, I will help you learn Python Sets. Python sets are an essential data structure that allows for efficient storage and manipulation of unordered collections of unique elements. We are going to cover some Python Sets concepts, exploring their definition, properties, and fundamental set operations. Additionally, we will discuss practical use cases, tips for learning Python sets, performance considerations, common mistakes to avoid, and a summary. In the end, I will also cover FAQs related to Python that can help you also prepare for an interview and clarify your doubt if any. Whether you are a beginner looking to learn more about sets or someone looking to refresh their knowledge, you’ve come to the right place. If you are new to Python Programming Language or need a refresher on its usage and benefits. I recommend you to go through my all about the Python programming language guide. If you are already familiar with Python and you just want to refresh the Python Sets understanding you are at the right place.
So let’s get started.!
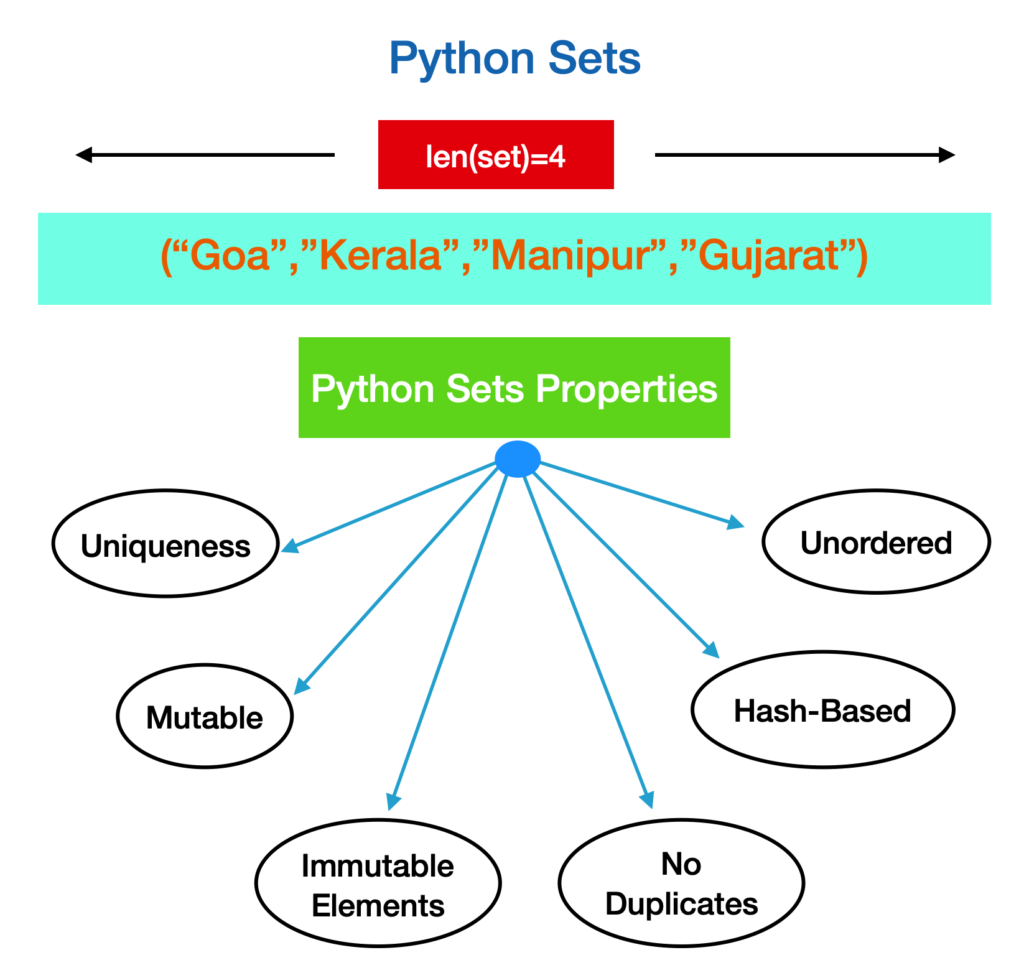
Understanding Python Sets
Sets in Python are unordered collections of unique elements that do not allow duplicates. Sets are one of the 4 data types that are used to store data in the Python programming language. The other Python data structure data types are List, Tuples, and Dictionary. Some important properties of Python sets are:
- Elements in a set are unique and immutable*.
(Immutable means that once the data structure is created, we cannot change, add or remove items to or from the Tuple) - Sets are mutable, allowing for modifications such as adding or removing elements.
(NOTE: Sets are kind of partially mutable, meaning you can’t change the set item but you can remove and add items. Also in the set, you can add immutable data types as an item in the set but not mutable data types like Dictionary or List) - Sets are unordered, meaning the elements have no specific order.
So as I mentioned, Set is a Python data structure. Python sets differ from other data structures in the following ways:
- Lists and tuples allow duplicates, while sets do not.
- Sets are unordered, unlike lists and tuples that maintain the insertion order of elements.
Creating and initializing sets in Python is straightforward. You can use the set() function or enclose elements within curly braces {}. Check out the below example. Feel free to take it and try all the above concepts, like adding an item, changing an item, or trying to change the order.
# Creating and Initializing sets using {} set_understanding1 = {"Goa","Kerala","Punjab","Delhi"} print(set_understanding1) print(len(set_understanding1)) ## Creating and Initializing sets using set() set_understanding2 = set(("Karnataka","Gujarat","Tamilnadu","Uttrakhand")) print(set_understanding2) # adding mutable datastructure to set set_understanding3 = set(("Karnataka",["Panji","Vasco"],"Tamilnadu","Uttrakhand")) print(set_understanding3) # adding immutable datastructure to set set_understanding4 = set(("Karnataka",("Panji","Vasco"),"Tamilnadu","Uttrakhand")) print(set_understanding4)
Basic Set Operations
One can perform several actions and operations on Python Set. I have explained some of the operations below:
Union operation: combining sets
The union operation combines two sets, resulting in a new set that contains all unique elements from both sets. One thing you need to note here is that performing union on two sets will not change the original set, instead it will create a completely new set. In python3 you can combine two sets (union operation) using either the union() method or the “|” operator. See the below code snippet to understand how it works.
#Union operation: combining sets # Creating and Initializing 2 sets set_1 = {"Goa",2,"Kerala",5,"Delhi"} set_2 = set((1,"Gujarat","Delhi",2)) # Using the union() method union_result = set_1.union(set_2) print(union_result) # Using the | operator for union union_result = set_1 | set_2 print(union_result)
Intersection operation: finding common elements
The intersection operation on sets returns a new set that contains the common elements between two or more sets. There are two ways to perform intersection operations on sets, the first is using the “&” operator and the second is the intersection() method. A code snippet is attached below for you to try intersection operation on your own. These operations are very useful in scenarios like Finding common elements, data analysis, finding mutual connections, and so on.
#Intersection operation: finding common elements # Creating and Initializing 2 sets set_1 = {"Goa",2,"Kerala",5,"Chhattisgarh", "Delhi"} set_2 = set((5,"Goa","Delhi",2,3,"Manipur")) # Using the intersection() method intersection_result = set_1.intersection(set_2) print(intersection_result) # Using the & operator for intersection intersection_result = set_1 & set_2 print(intersection_result)
Difference operation: elements in one set but not the other
This operation is the opposite of the intersection operation we learned above. The difference operation returns a new set with elements that exist in one set but not in the other. Whereas in interaction operations we saw that we get common elements. To perform a difference operation on Python set one can use the “-“ operator or the difference() method. Note that this operation will return an item present in the first set but not present in the second set. Such operations are used for filtering unique elements, excluding common elements, and so on. Feel free to explore more using the below code snippet.
#Difference operation: elements in one set but not the other set_1 = {"Goa",2,"Kerala",5,"Chhattisgarh", "Delhi"} set_2 = set((5,"Goa","Delhi",3,"Manipur")) # Using the - operator for difference difference_result = set_1 - set_2 print(difference_result) # Using the difference() method difference_result = set_1.difference(set_2) print(difference_result)
Symmetric Difference operation: elements found in either set, but not both
So if you were wondering how one can get unique elements from multiple sets. For that, you can use the symmetric difference operation. This operation returns a new set with elements that are present in either set but not in both. The “^” operator or the symmetric_difference() method can be employed for this operation. Such operations are used on sets to find exclusive elements, and in data analysis to identify data points that belong to only one group or category. Below is code demonstrating this operation.
#Symmetric Difference operation: elements found in either set, but not both set_1 = {"Goa",2,"Kerala",5,"Chhattisgarh", "Delhi"} set_2 = set((5,"Goa","Delhi",3,"Manipur")) # Using the ^ operator for symmetric difference symmetric_difference_result = set_1 ^ set_2 print(symmetric_difference_result) # Using the symmetric_difference() method symmetric_difference_result = set_1.symmetric_difference(set_2) print(symmetric_difference_result)
Advanced Set Operations
Apart from the basic set operations, there are advanced set operations that provide more insight into set manipulation:
Subset and Superset operations
A subset operation determines if one set is entirely contained within another set. For example, let’s consider two sets A & B. So in subset operation – set A is considered a subset of another set B if all the elements of A are also present in B. Note that the empty set is considered a subset of every set, including itself. In Python, you can use the issubset() method or the “<=” operator to check if one set is a subset of another.
A superset operation checks if one set contains another set entirely. For example, if we have two sets A and B. The set A is considered a superset of another set B if all the elements of B are also present in A. Note that the empty set is considered a superset of every set, including itself. In Python, to check if a set is a superset of another you can use the issuperset() method or the “>=” operator.
#Subset and Superset operations # Creating and Initializing 2 sets set_1 = {"Goa",2} set_2 = set((1,"Gujarat","Goa",2,"Kerala")) # Using the issubset() method is_subset = set_1.issubset(set_2) print(is_subset) # Using the <= operator for subset check is_subset = set_1 <= set_2 print(is_subset) # Using the issuperset() method is_superset = set_2.issuperset(set_1) print(is_superset) # Using the >= operator for superset check is_superset = set_2 >= set_1 print(is_superset)
Disjoint operation: checking for common elements
The disjoint operation checks if two sets have any common elements. If there are no common elements, the sets are considered disjoint. This operation is used to check the overlap of data and validate sets to ensure sets are unrelated or independent. To perform this operation you can use the isdisjoint() method. The method returns True if the sets are disjoint (i.e., they have no common elements) and False otherwise.
#Disjoint operation: checking for common elements set_1 = {"Goa","Jharkhand",9} set_2 = set((1,"Gujarat")) set_3 = {"Goa",2} # Using the isdisjoint() method to check for disjoint sets is_disjoint = set_1.isdisjoint(set_2) print(is_disjoint) # Using the isdisjoint() method with another set that shares an element is_disjoint = set_1.isdisjoint(set_3) print(is_disjoint)
Set comprehension: creating sets using conditional expressions
Set comprehension provides a short and clear way to create sets based on conditional expressions. It allows for filtering and transforming elements while creating sets. The general syntax for set comprehension is similar to list comprehension, but with curly braces {} to denote that a set is being created.
Syntax: new_set = {expression for the item in iterable if condition}
Use the below code to understand how it works. In the code, I am creating a set of squared odd numbers from a list.
#Set comprehension: creating sets using conditional expressions numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] # Set comprehension to create a set of squared even numbers squared_even_set = {x**2 for x in numbers if x % 2 != 0} print(squared_even_set) # Output: {1, 9, 81, 49, 25}
Practical Use Cases
Sets in Python have numerous practical applications for data manipulation and Data analysis. I have already mentioned a few of them above while covering set operations.
Removing duplicate elements from a list using sets
Python Sets can be used to efficiently remove duplicate elements. Let’s say you have a list of elements with few duplicates. One way to remove duplicates from that list is to convert the list to a set and then back to a list. This process ensures that only unique elements remain.
Finding unique values in a dataset using sets
In the case of data analysis and processing tasks, we need to deal with very large datasets. In order to quickly identify and extract unique values we can use the one or combination of above mentioned sets operations.
Efficient membership testing with sets
Sets offer fast membership testing, allowing you to check whether an element exists in a set or not. This can significantly improve the performance of operations involving membership testing.
Performing set operations on large datasets
Sets have the ability to quickly eliminate duplicates and optimize computation time. That is the reason Sets are particularly efficient when performing set operations on large datasets.
Tips for Learning Python Sets
Below are the tips I used when I was learning Python Sets. You can try the below tips:
Understand set theory basics
Having a basic understanding of set concepts. This can enhance your ability to understand set operations and their applications.
Practice with small examples
I have already attached code snippets to explain concepts and operations with examples. So start by experimenting with those small examples to gain familiarity with Python set operations. This approach can help solidify your understanding and build confidence.
Leverage built-in Python functions for set operations
I always prefer this. Python3 provides lots of built-in functions for set operations. Like union(), intersection(), difference() and many others. Utilize these functions to simplify your code and improve efficiency.
Utilize Python set methods effectively
Python offers various set methods, including add(), remove(), and clear(). Familiarize yourself with these methods to perform specific operations on sets effectively.
Performance Considerations
In any code i.e. requirement meeting or problem solving code logic solution. Understanding the performance of Python Sets operations is crucial. Especially when dealing with large datasets. Consider the following aspects:
The time complexity of set operations
Each set operation has a specific time complexity. For example, the union operation has a time complexity of O(len(s1) + len(s2)). Knowing these complexities helps in optimizing your code.
Optimizing set operations for large datasets
In practical scenarios, we mostly deal with large datasets. You can leverage techniques like indexing, indexing subsets, or using frozen sets, (i.e. immutable sets) to optimize set operations.
Memory considerations when using sets
While sets provide efficient storage for unique elements, they require additional memory compared to other data structures due to their internal implementation.
Common Mistakes and Pitfalls
To avoid unnecessary errors and pitfalls when working with sets, be aware of the following common mistakes:
Incorrectly modifying set elements during iteration
It is better to create a new set or use a temporary set to store modified elements. Modifying set elements during iteration can lead to unexpected results.
Overlooking type restrictions in set operations
Set operations require elements to be hashable. Be mindful of not including unhashable objects like lists or dictionaries in sets.
Neglecting to convert data types for set operations
Ensure proper conversion when necessary to avoid errors and unexpected results. Especially when performing set operations with different data types,
Summary
To summarize, Python Sets are valuable data structures that allow for efficient storage and manipulation of unique elements. Once you have understood the basic and advanced set operations, it provides great flexibility in data manipulation and analysis. Python developers can enhance their code’s performance by leveraging the advantages of sets, such as removing duplicates, finding unique values, and efficient membership testing. Remember to consider performance considerations, avoid common mistakes, and understand optimal use cases for sets to maximize their potential in code logic.
You can get to know more about Python Sets from Official Python Set Document.
If you have any questions, need further guidance, or seek consulting services, feel free to get in touch with me. You can reach out to me through this Contact Form to discuss your specific needs and requirements.
Frequently Asked Questions (FAQs)
Here are some frequently asked questions related to Python sets:
What is the difference between a set and a list in Python?
Sets are unordered collections of unique elements, while lists allow duplicate elements and maintain their insertion order.
Can sets contain mutable objects in Python?
No, sets can only contain immutable objects since their elements must be hashable.
How can I concatenate multiple sets in Python?
To concatenate multiple sets, you can use the union() method or the “|” operator.
How can I check if a set is empty in Python?
You can use the len() function to check if the length of a set is zero, which indicates an empty set.
Is the order of elements preserved in a set?
No, sets in Python are unordered, meaning the elements do not follow a specific order.
Can I perform mathematical operations with sets in Python?
Yes, Python provides various mathematical operations for sets, such as union, intersection, and difference, allowing for set manipulations based on set theory principles.
I recommend you improve your learning and knowledge of Python Set through more practice. For that, I would suggest you try out questions covered in Python Sets with Excerice and 30 Questions to Test Your Knowledge of Python Sets.
Hope I have made it easy to understand the Python Sets and its basic operations. If you like this article and think it was easy to understand and might help someone you know, do share it with them. Thank You! See you soon.
If you have any questions or comments feel free to reach me at.
Checkout out other Python concept covered in Python Tutorial